I started out with the Arduino platform and developed a lot with it. The Atmel processors that come with them are great and I especially love the small form-factor Arduino Pro Micro boards for small projects since it not only has its own USB interface, but also a separate, additional UART unit to allow communication with peripherals, and the usual niceties of Arduino’s integrated programming environment.
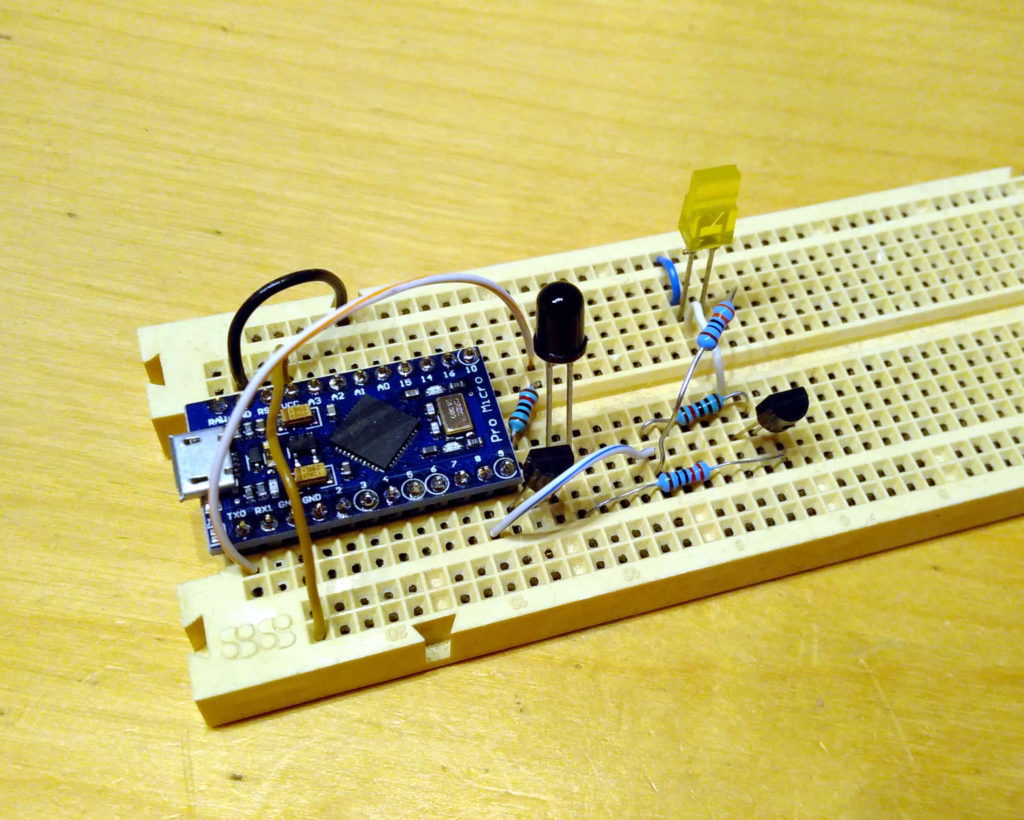
However, as nice as the Arduino platform is, it is relatively bulky and expensive. The original Pro Micro from Sparkfun costs USD 20 (or EUR if imported) and even the cheaper clones cost 5 EUR minimum (the reliable ones that is). This made me think about alternatives and I decided to try out PIC micro controllers from Microchip for a more integrated solution.
These PIC series of microcontrollers come in a huge (huge!) variety and can cost anything between a few cents up to tens of Euros per chip and they have a ginormous range of features – on a single chip! Other than the Atmel processors they typically are self sufficient enough to run on their own, just with a power supply connected. They come with on-board capacitive clock generators, UART controller, PWM, memory, EEPROMs,… you can select what you need using Microchip’s quirky and slow, but quite handy part selector.
I bought PIC16F1705s and PIC16F1709s as my main prototyping platform. These have a decent set of features like analog comparators, counters, timers, UART, I2C, features I regularly use to interface with other analog or digital peripherals.
Programming PICs
Programming PICs is a big fat minus point for this type of microcontroller. The Arduino platform has everything nicely solved on-board, but since PIC microcontrollers come only as a single chip, one needs to manually attach a suitable programmer. I tried to make my own and it works for a single chip type, but I ended up to just buy a PICKit 3 in order to be able to easily program all of my chips. The first step to program a PIC is to install MPLAB-X which on its own is easy enough. Additionally, you need to select the correct compiler you want to use, and match it to the chip you want to program. The PIC board then needs to be awkwardly connected to the PICkit like show in the following image:

Quite awkward, right? Anyway, this is not the only hurdle to use the small formfactor PICs. The code for a simple “blink LED” is quite cryptic on its compared to an Arduino counter-part:
// ... boiler plate ...
// This will "blink" output RC0
void main() {
TRISC = 0b00000000;
while(1) {
LATC = 0b00000001;
__delay_ms(1000);
LATC = 0b00000000;
__delay_ms(1000);
}
}
libpic170x – making it more verbose
I think that this is cryptic, stupid and can be improved upon. At least for easing the prototyping phase of a project. The cryptic names for registers that one needs to use differ across microcontrollers and the number of cryptic names one needs to use increases considerably when attempting to control the PIC’s on-board UART, timers, or other devices. Therefore, I started the project libpic170x, available on GitHub. Using the library, the blink program becomes less cryptic:
void main() {
pin_set_pin_mode(PIN_RC0, true);
pin_set_output(PIN_RC0, false);
while (1) {
pin_set_output(
PIN_RC0,
!pin_get_input(PIN_RC0));
__delay_ms(1000);
}
}
I am adding new functions to the library as I am using new features of the PIC, so feel free to check out the library. The most useful feature, to date is the Serial library( which is not yet included in an official release, probably coming soon).
Please note though, that the library, while convenient, can add a lot of overhead when in comes to execution speed. Writing registers like the latch registers (LATC) directly for switching IO states translates to just a few lines of machine code. In order to make functions like pin_set_pin_mode safe to use, they contain a lot of checks which make the library comparatively slow – my best guess about the overhead is a slow-down of a factor of 30-40. The utility when controlling GPIO pins therefore is limited, but for higher-level functions like controlling the UART, the library is very capable.
Future development
I intend to keep the library updated whenever I use a new feature of the PIC. Currently, the library only works for the PIC16(L)F170x series of chips, because I do not have many other PIC chips, but it is possible that it is compatible with other chips as well. I intend to keep the documentation updated so that everybody who wants to try it, can use the library with a low barrier to entry.